Flutter框架结构
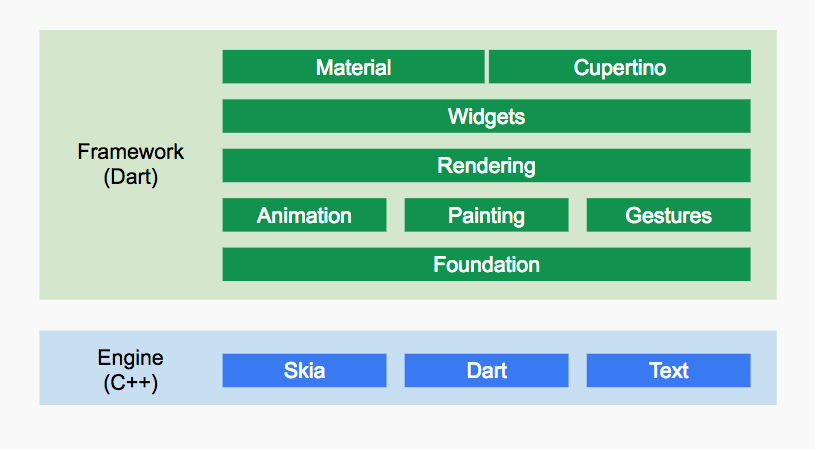
底下两层(Foundation和Animation、Painting、Gestures被合并为一个dart UI层,它是Flutter引擎暴露的底层UI库,提供动画、手势及绘制能力。
Rendering层,这一层是一个抽象的布局层,它依赖于dart UI层,Rendering层会构建一个UI树,当UI树有变化时,会计算出有变化的部分,然后更新UI树,最终将UI树绘制到屏幕上,这个过程类似于React中的虚拟DOM。Rendering层可以说是Flutter UI框架最核心的部分,它除了确定每个UI元素的位置、大小之外还要进行坐标变换、绘制(调用底层dart:ui)。
Widgets层是Flutter提供的的一套基础组件库,在基础组件库之上,Flutter还提供了 Material 和Cupertino两种视觉风格的组件库。而我们Flutter开发的大多数场景,只是和这两层打交道。
primarySwatch 和 primaryColor 的区别
1 2 3 4 5 6 7 8
| Widget build (BuildContext context) { return MaterialApp( title: 'myApp', theme: ThemeData( primaryColor: Colors.white // primarySwatch ) ) }
|
使用primaryColor可以Colors.white,使用primarySwatch不可以设置白色和黑色,primarySwatch中的颜色是调用MaterialColor这种颜色类
listView
1 2 3 4 5 6 7 8 9 10 11 12
| Widget _buildSuggestions() { return ListView.builder( padding: EdgeInsets.all(16.0), itemCount: 100, itemBuilder: (BuildContext context, i) { //itemBuilder callback if (i.isOdd) { return Something(); } return Another() } ) }
|
异步编程
与js一样,dart支持单线程执行,js中Promise对象表示异步操作的最终结果,dart中用Future对象来处理
1 2 3 4 5 6
| _getIpAddress() { final url = 'http://www.google.com'; HttpRequest.reqeust(url).then((value) => { print(json.decode(value.responseText)['origin']); }).catchError((error) => print(error)) }
|
async函数返回Future,await等待Future
1 2 3 4 5 6
| _getIpAddress() { final url = 'http://www.google.com'; var request = await HttpRequest.reqeust(url); String ip = json.decode(request.responseText)['origin']; print(ip); }
|
布局
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| Contianer( child: Center( child: Container( child: Text('demo'), decoration: BoxDecoration( // color, borderRadius, boxShadow, shape 通过BoxDecoration装饰 color: Colors.red, borderRadius: BorderRadius.all( Radius.circular(8.0) ), boxshadow: <BoxShadow>[ BoxShadow( color: Color(0xcc000000), offset: Offset(0.0, 2.0) ), BoxShadow( color: Color(0000000000), offset: Offset(0.0, 2.0) ) ], shape: BoxShape.circle ), padding: EdgesInsets.all(16.0), width: 240.0 ) ) )
|
- Positioned widget and Stack widget
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| Container( child: Stack( children: [ Positioned( child: Container( child: Text('demo'), decoration: BoxDecoration( color: Colors.red ) ), left: 24.0, top: 24.0 ) ] ), width: 320.0, height: 320.0 )
|
- Transform widget and Scaling widget
1 2 3 4 5 6 7 8 9 10 11 12
| Container( child: Transform( child: Container( child: Text('demo') ), alignment: Alignment.center, transform: Matrix4.identity()..rotateZ(15 * 3.1415926 / 180) // rotate transform: Matrix4.identity()..scale(1.5) // scale ), width: 320.0, height: 320.0 )
|
1 2 3 4 5 6 7 8 9 10 11
| Container( child: Container( child: Text( child: Text('demo'), overflow: TextOverflow.ellipsis, maxLines: 1 ), ), width: 320.0, height: 320.0 )
|
GestureDetector 处理手势
1 2 3 4 5 6 7 8 9 10 11 12 13
| class MyButton extends StatelessWidget { @override Widget build (BuildContext context) { return GestureDetector( // IconButton等使用GestureDetector提供onPressed回调 onTap: () { // onLongPress... print('tapped') }, child: Container( child: Text('demo') ) ) } }
|
final const var static in Dart
static: 表示一个成员属于类而不是对象,修饰成员
final: 必须初始化,且值不可变,编译时不能确定值,修饰变量 // final list = [1,2,3]; list[0] = 4; => [4,2,3]
const: 编译时可确定,并且不能修改 // var list = const [1,2,3]; list[0] = 4; error
typedef 类型定义,通过用来检查函数类型
1 2 3 4 5
| typedef int Compart(int a, int b); int sort(int a, int b) => a - b; main() { assert(sort is Compare); // True }
|